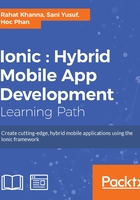
$http services
In web technologies, the best way to interact with any web service is through Ajax requests. As Ionic Framework is a Hybrid Mobile framework based on web technologies, it utilizes the power of Ajax to wire up Ionic Apps with any web services.
$http
is an in-built Angular service that is used as an abstraction for native JavaScript Ajax calls. The $http
service has some high-level methods exposed to make HTTP requests using different HTTP methods such as POST
, GET
, PUT
, and so on.
There are different signatures for different methods, but the response for all the methods is exactly the same. All the methods in the $http
service are based on the promise
objects, which help in registering success and error callbacks that receive data at a later point in time as these requests are asynchronous in nature.
The two most important methods are .get
and .post
whose usage is as follows:
$http.get('/api/url/resource') .then(function(response){ // this is success callback },function(errorResponse) { // this is error callback });
The GET
request method takes only one argument, the URL for the request, and registers two callbacks with the method of the promise
object returned:
$http.post('/api/url/resource',{data:'custom data'}) .then(function(response){ // this is success callback },function(errorResponse) { // this is error callback });
The POST
request method takes two arguments, the URL for the request and the data to be sent in the body of the post request.
The other methods available that have a signature exactly like the GET
method are as follows:
$http.put
$http.head
$http.delete
$http.jsonp
$http.patch
There are default headers sent along with each HTTP request. Some web services require us to send custom headers for authorization or content-type for which the $http
service exposes a provider to set custom default headers. We can use the provider to set up default headers in the config
phase of the Ionic App. We can also use the $http
object to set default headers in the run
block or any controller/service:
module.config(function($http) { $http.defaults.headers.common.Content-Type = 'application/json'; }); module.run(function($http) { $http.defaults.headers.post.Authorization = 'Basic sdkJKHSmd' });
The response object
The response
object passed as an argument to the callback functions contains the following properties:

The response
object can be used to extract the data sent by the server and process it.
The $http constructor method
Another way to make an Ajax request is by using the $http
constructor method and passing the request configuration object directly. For example:
var req = { method: 'POST', url: '/api/url/resource', headers: { 'Content-Type': 'plain/text' }, data: { objectName: 'Value' }, cache: true } $http(req).then(function(){...}, function(){...});
The $http
service also supports caching by passing the cache
property to the configuration object and setting it to true
. Angular stores the response temporarily to the $cacheFactory
object and serves the same request with a response from there.