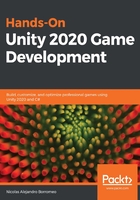
GameObjects and components
We talked about our project being composed of Assets, and that a Scene (a specific type of Asset) is composed of GameObjects; so, how can we create an object? Through the composition of components.
In this section, we will cover the following concepts related to components:
- Components
- Manipulating components
Components
A component is one of several pieces a Game Object can be made of; each one is in charge of different features of the object. There are several components that solve different tasks, such as playing a sound, rendering a mesh, applying physics, and so on, and even if Unity has a large number of components, we will eventually need to create custom components, sooner or later. In the following screenshot, you can see what Unity shows us when we select a Game Object:

Figure 3.13 – The Inspector panel
In the previous screenshot, we can see the Inspector panel, and if we needed to guess what it does, right now we could say it is showing all the properties of the selected object and that we can configure them to change the behavior of the object, such as the position and rotation, whether it will project shadows or not, and so on. That is true, but we are missing a key element: those properties don't belong to the object; they belong to the components of the object. We can see some titles in bold before a group of properties, such as Transform and Box Collider, and so on. Those are the components of the object.
In this case, our object has a Transform, a Mesh Filter, a Mesh Renderer, and a Box Collider component, so let's review each one of those. Transform just has location information, such as the position, rotation, and scale of the object, and by itself it does nothing—it's just a point in our game—but as we add components to the object, that position starts to have more meaning. That's because some components will interact with Transform and other components, each one affecting the other.
An example of that would be the case of Mesh Filter and Mesh Renderer, both of those being in charge of rendering a 3D model. Mesh Renderer will render the mesh specified in the Mesh Filter in the position specified in the Transform component, so Mesh Renderer needs to get data from those other components and can't work without them. Another example would be the Box Collider. This represents the physical shape of the object, so when the physics calculates collisions between objects, it checks whether that shape is colliding with other shapes based on the position specified in the Transform.
We don't want to explore physics and rendering right now. The takeaway from this section is that a GameObject is a collection of components, each component adding a specific behavior to our object, and each one interacting with the others to accomplish the desired task. To further reinforce this, let's see how we can convert a cube into a sphere that falls using physics.
Manipulating components
The tool to edit an object's components is the Inspector. It not only allows us to change components' properties, but also lets us add and remove components. In this case, we want to convert a cube to a sphere, so we need to change several aspects of those components. We can start by changing the visual shape of the object, so we need to change the rendered model or Mesh. The component that specifies the Mesh to be rendered is the MeshFilter component. If we look at it, we can see a Mesh property that says Cube and that has a little circle with a dot to its right.
Important note
If you don't see any property such as the Mesh we just mentioned, try to click the triangle at the left of the component's name. Doing this will expand and collapse all the component's properties. This is illustrated in the following screenshot:

Figure 3.14 – Disabling a component
If we click it, the Select Mesh window will pop up, allowing us to pick several Mesh options; so, in this case, select the Sphere component. In the future, we will add more 3D models to our project so that the window will have more options. The mesh selector is shown in the following screenshot:

Figure 3.15 – Mesh selector
Okay—it looks like a sphere, but will it behave like a sphere? Let's find out. In order to do so, we can add a Rigidbody component to our sphere, which will add physics to it. In order to do so, we need to click the Add Component button at the bottom of the Inspector. It will show a Component Selector window with lots of categories, and, in this case, we need to click on the Physics category. The window will show all the Physics components, and there we can find the Rigidbody. Another option would be to type Rigidbody in the search box at the top of the window. The following screenshot illustrates how to add a component:

Figure 3.16 – Adding components
If you hit the Play button in the top-middle part of the editor, you can test your sphere physics using the Game panel. That panel will be automatically focused when you hit Play and will show you how the player will see the game. One problem that can happen here is that maybe you won't see anything, and that can happen if the game camera is not pointing to where our sphere is located. The playback controls are shown in the following screenshot:

Figure 3.17 – Playback controls
Here, you can just use the Transform Gizmo to rotate and position your camera in such a way that it looks at our sphere. While you are moving, you can check the little preview in the bottom-right part of the scene window to check out the new camera perspective. Another alternative would be to select the camera in the Hierarchy and use the shortcut Ctrl + Shift + F (or command + Shift + F on a Mac). The camera preview is shown in the following screenshot:

Figure 3.18 – Camera preview
Now, to test whether Physics collisions are executing properly, let's create a cube, scale it until it has the shape of a ramp, and put that ramp below our sphere, as follows:

Figure 3.19 – Ball and ramp objects
If you hit Play now, you will see the sphere colliding with our ramp, but in a strange way. It looks like it's bouncing, but that's not the case. If you expand the Box Collider component of our sphere, you will see that even if our object looks like a sphere, the green box gizmo is showing us that our sphere is actually a box in the Physics world, as illustrated in the following screenshot:

Figure 3.20 – Object with sphere graphic and box collider
Nowadays, video cards can handle rendering highly detailed models (with high polygon counts), but the Physics system is executed in the central processing unit (CPU) and it needs to do complex calculations in order to detect collisions. To get decent performance in our game (at least 30 frames per second (FPS)) the Physics system works using simplified collision shapes that may differ from the actual shape the player sees on the screen. That's why we have Mesh Filter and the different types of Collider components separated—one handles visual shape and the other the physics shape.
Again, the idea of this section is not to deep pe into those Unity systems, so let's just move on for now. How can we solve this? Simple: by modifying our components! In this case, BoxCollider can just represent a box shape, unlike MeshFilter, which supports any shape. So, first, we need to remove it by right-clicking the component's title and selecting the Remove Component option, as illustrated in the following screenshot:

Figure 3.21 – Removing components
Now, we can again use the Add Component menu to select a Physics component, this time selecting the Sphere Collider component. If you look at the Physics components, you will see other types of colliders that can be used to represent other shapes, but we will look at them later in Chapter 15, Physics Collisions and Health System. The Sphere Collider component can be seen in the following screenshot:

Figure 3.22 – Adding a Sphere Collider component
So, if you hit Play now, you will see that our sphere not only looks like a sphere, but also behaves as one. Remember: the main idea of this section of the book is understanding that in Unity you can create whatever object you want just by adding, removing, and modifying components, and we will be doing a lot of this throughout the book.
Now, components are not the only thing needed in order to create objects. Complex objects may be composed of several sub-objects, so let's see how that works.