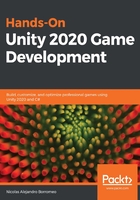
Manipulating scenes
A scene is one of the several kinds of files (also known as Assets) in our project. A scene can mean different things according to the type of project or the way a company is used to working, but the most common use case is to separate your game into whole sections, the most common ones being the following:
- Main Menu
- Level 1, Level 2, Level 3, …, Level N
- Victory Screen, Lose Screen
- Splash Screen, Loading Screen
In this section, we will cover the following concepts related to scenes:
- The purpose of a scene
- The Scene View
- Our first GameObject
- Navigating the Scene View
- Manipulating GameObjects
The purpose of a scene
The idea of separating your game into scenes is that you will process and load just the data needed for the scene; so, if you are in the Main Menu you will have only the textures, music, and objects that that particular scene needs—there's no need to have the Level 10 Boss loaded in random-access memory (RAM) if you don't need it right now. That's why loading screens exist, just to fill the time between unloading the Assets needed in one scene and loading the ones needed in another. Maybe you are thinking that open-world games such as Grand Theft Auto (GTA) don't have loading screens while you roam around in the world, but they are actually loading and unloading chunks of the world in the background as you move, and those chunks are different scenes that are designed to be connected to each other.
The difference between the Main Menu and a regular level scene are the objects (also known as GameObjects) they have. In a menu, you will find objects such as a background, music, buttons, and logos, and in a level you will have the player, enemies, platforms, health boxes, and so on. So, it is up to you and the GameObjects you put in the scene to decide what that scene means for your game.
But how can we create a scene? Let's start with the Scene View.
The Scene View
When you open a Unity project, you will see the Unity Editor. It will be composed of several windows or panels, each one helping you to change different aspects of your game. In this chapter, we will be looking at the windows that help you author scenes. The Unity Editor is shown in the following screenshot:

Figure 3.1 – Unity Editor
If you have ever programmed any kind of application before, you are probably used to having a starting function, such as Main, where you start writing code to create several objects needed for your app, and if we are talking about games, you probably create all the objects for the scene here. The problem with this approach is that in order to ensure all objects are created properly, you will need to run the program to see the results, and if something is misplaced you will need to manually change the coordinates of the object, which is a slow and painful process. Luckily, in Unity, we have the Scene View, an example of which is shown in the following screenshot:

Figure 3.2 – Scene View
This window is an implementation of the classic WYSIWYG (What You See Is What You Get) concept. Here, you can create objects and place them all over the scene, all through a scene previsualization where you can see how the scene will look when you hit Play. But before learning how to use this scene, we need to have an object in the scene, so let's create our first object.
Our first GameObject
The Unity Universal Render Pipeline (URP) template comes with a construction site test scene, but let's create our own empty scene to start exploring this new concept. To do that, you can simply use the File | New Scene menu options to create an empty new scene, as illustrated in the following screenshot:

Figure 3.3 – Creating a new scene
We will learn several ways of creating GameObjects throughout the book, but now, let's start using some basic templates Unity provides us. In order to create them, we will need to open the GameObject menu at the top of the Unity window, and it will show us several template categories, such as 3D Object, 2D Object, Effects, and so on, as illustrated in the following screenshot:

Figure 3.4 – Creating a cube
Under the 3D Object category, we will see several 3D primitives such as Cube, Sphere, Cylinder, and so on, and while using them is not as exciting as using beautiful downloaded 3D models, remember that we are prototyping our level, also known as gray-boxing. This means that we will use lots of prototyping primitive shapes to model our level so that we can quickly test it and see whether our idea is good enough to start the complex work of converting it to a final version.
I recommend you pick the Cube object to start with because it is a versatile shape that can represent lots of objects. So, now that we have a scene with an object to edit, the first thing we need to learn to do with the scene view is to navigate through the scene.
Navigating the Scene View
In order to manipulate a scene, we need to learn how to move through it to view the results from different perspectives. There are several ways to navigate it, so let's start with the most common one: the first-person view. This view allows you to move through the scene using first-person-shooter-like navigation, using the mouse and the WASD keys. To navigate like this, you will need to press and hold the right mouse button, and while doing so, you can move the mouse to rotate the camera and press the WASD keys to move it. You can also press Shift to move faster and press the Q and E keys to move up and down.
Another common way of moving is to click an object to select it (the selected object will have an orange outline), and then press the F key to focus it, making the Scene View camera immediately move into a position where we to look at that object more closely. After that, we can press and hold the left Alt key and the left mouse button, and start moving the mouse to "orbit" around the object and see different angles to check that every part of it is properly placed, as demonstrated in the following screenshot:

Figure 3.5 – Selecting an object
Now that we can move freely through the scene, we can start using the Scene View to manipulate GameObjects.
Manipulating GameObjects
Another use of the Scene View is to manipulate the locations of objects. In order to do so, we first need to select an object, and then press the Y key on the keyboard or the sixth button to the right in the top-left corner of the Unity Editor, shown in the following screenshot:

Figure 3.6 – Changing the transformation tool
This will show what is called the Transform Gizmo over the selected object, which allows us to change the position, rotation, and scale of the object, as illustrated in the following screenshot:

Figure 3.7 – Transform Gizmo
Let's start translating the object, which is accomplished by dragging the red, green, and blue arrows inside the Gizmo´s sphere. While you do this, you will see how the object will be moving along the selected axis. An interesting concept to explore here is the meaning of the color of those arrows. If you pay attention to the top-right area of the Scene View, you will see an axis Gizmo that serves as a reminder of those colors' meaning, as illustrated in the following screenshot:

Figure 3.8 – Axis Gizmo
Computer graphics use the classic 3D Cartesian coordinate system to represent objects' locations. The red color is associated with the x axis of the object, green with the y axis, and blue with the z axis. But what does each axis mean? If you come from another 3D authoring program, this can be different, but in Unity, the z axis (blue) represents the Forward Vector, which means that the arrow is pointing along the front of the object; the x axis is the Right Vector, and the y axis represents the Up Vector. Consider that those axes are local, meaning that if you rotate the object, they will change the direction they face because the orientation of the object changes the way the object is facing. Unity can show those axes in global coordinates if necessary, but for now, let's stick with local.
In order to be sure that we are working with local coordinates, make sure Local mode is activated, as shown in the following screenshot:

Figure 3.9 – Switching between pivot and local coordinates
If you see Global instead of Local as the right button, just click it and it will change. By the way, try to keep the left button as Pivot. If it says Center, just click it to change it.
I know—we are editing a cube, so there is no clear front or right side, but when you work with real 3D models such as cars and characters, they will certainly have those sides, and they must be properly aligned with those axes. If by any chance in the future you import a car into Unity and the front of the car is pointing along the red axis (X), you will need to fix that because our future moving code will rely on that convention, but let's keep that for later.
Now, let's use this Transform Gizmo to rotate the object using the three colored circles around it. If you click and drag, for example, the red circle, you will rotate the object along the x rotation axis. Here is another interesting tip to consider. If you want to rotate the object horizontally, based on the color-coding we previously discussed, you will probably pick the x axis—the one that is used to move horizontally—but, sadly, that's wrong.
A good way to look at rotation is like the accelerator of a bike: you need to take it and roll it. If you rotate the x axis like this, you will rotate the object up and down. So, in order to rotate horizontally, you would need to use the green circle or the y axis. The process is illustrated in the following screenshot:

Figure 3.10 – Rotating an object
Finally, we have scaling, which is done through the colored cubes on the outer side of the Transform Gizmo sphere. If you click and drag those, you will see how our cube is stretched over those axes, allowing you to change the size of the object. Also, you will have a gray cube in the center of the gizmo that allows you to change the size of the object uniformly along all the axes. The process is illustrated in the following screenshot:

Figure 3.11 – Scaling an object
Remember that scaling objects is usually a bad practice in many cases. In the final versions of your scene, you will use models with the proper size and scale, and they will be designed in a modular way so that you can plug them one next to the other. If you scale them, several bad things can happen, such as textures being stretched and becoming pixelated, and modules that no longer plug properly. There are some exceptions to this rule, such as placing lots of instances of the same tree in a forest and changing its scale slightly to simulate variation, and, in the case of gray-boxing, it is perfectly fine to take cubes and change the scale to create floors, walls, ceilings, columns, and so on, because in the end, those cubes will be replaced with real 3D models.
CHALLENGE
Create a room composed of a floor, three regular walls, and a fourth wall with a hole for a door (three cubes). In the following screenshot, you can see how it should look:

Figure 3.12 – Room task finished
Now that we can edit an object's location, let's see how we can edit all its other aspects.