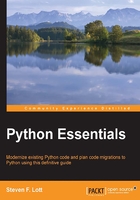
The Python namespace concept
We've already seen two applications of the Python namespace. When we assign variables at the >>>
prompt, we're introducing the variable into the global namespace. When we import a module, the module creates its own namespace within the global namespace.
That's why we can then use qualified names like math.sqrt()
to refer to objects inside the module's namespace.
When we look at functions and class definitions, we'll see additional use, of namespaces. In particular, when evaluating a function or a class method, a local namespace is created, and all variables are part of that local namespace. When the function evaluation finishes (because of an explicit return
statement or the end of the indented block,) the local namespace is dropped, removing all local variables and reducing the reference count on all objects assigned to those local variables.
Additionally, the types
module includes the SimpleNamespace
class. An instance of this class allows us to build a complex object without a formal class definition. Here's an example:
>>> from types import SimpleNamespace >>> red_violet= SimpleNamespace(red=192, green=68, blue=143) >>> red_violet namespace(blue=143, green=68, red=192) >>> red_violet.blue 143
We've imported the SimpleNamespace
class. We created an instance of that class, assigning three local variables, red
, green
, and blue
, that are part of the new SimpleNamespace
object. When we examine the object as a whole, we see that it has three internal variables.
We can use syntax like red_violet.blue
to see the blue
variable inside the red_violet
namespace.
The argparse
module is used by command-line programs to parse the command-line arguments. This module also contains a Namespace
class definition. An instance of Namespace
is used to collect the various arguments parsed from the command line. An application can set additional variables in the Namespace
object to handle particularly complex parsing and configuration issues.
Globals and locals
When we use a variable name in an expression, Python searches two namespaces to resolve the name and locate the object to which it refers. First, it checks the local namespace. If the name is not found, it will check the global namespace. This two-step search will ensure that local variables used inside a function or class method are used before global variables with the same name.
When working from the >>>
prompt using the REPL, we can only create and use global variables. Further examples will have to wait until Chapter 7, Basic Function Definitions.
When we use the locals()
and globals()
functions at the >>>
prompt, we can see that they have the same results. At the >>>
prompt, and at the top-level of a script file, the local namespace is the global namespace. When evaluating a function, however, the function works in a separate, local namespace.