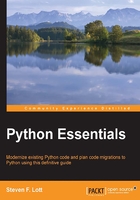
The print() function
When working with Python's REPL, we can enter an expression and Python prints the result. In other contexts, we must use the print()
function to see results. The print()
function implicitly writes to sys.stdout
, so the results will be visible on the console where we ran the Python script.
We can provide any number of expressions to the print()
function. Each value is converted to a string using the repr()
function. The strings are combined with a default separator of ' '
and printed with a default line ending of '\n'
. We can change the separator and line ending characters. Here are some examples of this:
>>> print("value", 355/113) value 3.1415929203539825 >>> print("value", 355/113, sep='=') value=3.1415929203539825 >>> print("value", 355/113, sep='=', end='!\n') value=3.1415929203539825!
We've printed a string and the floating-point result of an expression. In the second example, we changed the separator string from a space to '='
. In the third example, we changed the separator string to '='
and the end-of-line string to '!\n'
.
Note that the sep
and end
parameters must be provided by name; these are called keyword arguments. Python syntax rules require that keyword argument values are provided after all of the positional arguments. We'll examine the rules in detail in Chapter 7, Basic Function Definitions.
We can use ,
as a separator to create simple comma-separated values (CSV) files. We can also use \t
to create a kind of CSV file with a tab character as the column separator. The csv
library module does an even more complete job of CSV formatting, specifically including proper escapes or quoting for data items which contain the separator character.
To write to the standard error file, we'll need to import the sys
module, where that object is defined. For example:
import sys print("Error Message", file=sys.stderr)
We've imported the sys
module. This contains definitions of sys.stderr
and sys.stdout
for the standard output files. By using the file=
keyword parameter, we can direct a specific line of output to the stderr
file instead of the default of stdout
.
This can work well in a script file. Using the standard error file doesn't look very interesting at the REPL prompt since, by default, both standard output and standard error go to the console. Some IDE's will color-code the standard error output. We'll look at many ways to open and write to other files in Chapter 10, Files, Databases, Networks, and Contexts.