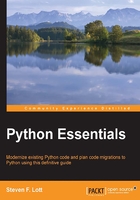
Using the tuple collection
The tuple
is one of the simplest collections available in Python. It is one of the many kinds of Python sequences. A tuple has a fixed number of items. For example, we might work with (x, y) coordinates or (r, g, b) colors. In these cases, the number of elements in each tuple is fixed by the problem domain. We don't want the flexibility of a collection that can vary in length.
Generally, we'll include ()
around a tuple
to set it apart from the surrounding syntax. This isn't always required; Python creates tuple
objects implicitly in some common contexts. However, it is always a good idea. If we write an assignment statement like this:
a = 2, 3
This statement will implicitly create a 2-tuple, (2, 3)
, and assign the object to the variable a
.
The tuple
class is part of Python's family of Sequence
classes; we can extract the items of a tuple
using their positional indices. The str
and byte
classes are also examples of Sequence. In addition to simple index values, we can use slice notation to pick items from a tuple
.
The value ()
is a zero-length tuple. To create a singleton tuple, we must use ()
and include a ,
character: this means that (12,)
is a singleton tuple. If we omit the ,
character we've written an expression, not a singleton tuple.
A trailing comma is required for a singleton tuple. An extra comma at the end of a tuple is quietly ignored everywhere else: (1, 1, 2)
is equal to (1, 1, 2,)
.
The tuple
class offers only two method functions: count()
and index()
. We can count the number of occurrences of a given item in a tuple
, and we can locate the position of an item in a tuple
.