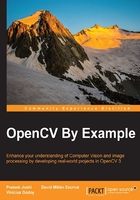
Reading/writing images
After the introduction of this matrix, we are going to start with the basics of the OpenCV code. Firstly, we need to learn how to read and write images:
#include <iostream> #include <string> #include <sstream> using namespace std; // OpenCV includes #include "opencv2/core.hpp" #include "opencv2/highgui.hpp" using namespace cv; int main( int argc, const char** argv ) { // Read images Mat color= imread("../lena.jpg"); Mat gray= imread("../lena.jpg", 0); // Write images imwrite("lenaGray.jpg", gray); // Get same pixel with opencv function int myRow=color.cols-1; int myCol=color.rows-1; Vec3b pixel= color.at<Vec3b>(myRow, myCol); cout << "Pixel value (B,G,R): (" << (int)pixel[0] << "," << (int)pixel[1] << "," << (int)pixel[2] << ")" << endl; // show images imshow("Lena BGR", color); imshow("Lena Gray", gray); // wait for any key press waitKey(0); return 0; }
Let's try to understand this code:
// OpenCV includes #include "opencv2/core.hpp" #include "opencv2/highgui.hpp" using namespace cv;
First, we have to include the declarations of the functions that we need in our sample. These functions come from core (basic image data handling) and high-gui (the cross-platform I/O functions provided by OpenCV are core
and highui
. The first includes the basic classes, such as matrices, and the second includes the functions to read, write, and show images with graphical interfaces).
// Read images Mat color= imread("../lena.jpg"); Mat gray= imread("../lena.jpg", 0);
The imread is the main function used to read images. This function opens an image and stores the image in a matrix format. The imread
function accepts two parameters: the first parameter is a string that contains the image's path, and the second parameter is optional and, by default, loads the image as a color image. The second parameter allows the following options:
CV_LOAD_IMAGE_ANYDEPTH
: If set to this constant, returns a 16-bit/32-bit image when the input has the corresponding depth; otherwise, theimread
function converts it to an 8-bit imageCV_LOAD_IMAGE_COLOR
: If set to this constant, always converts the image to colorCV_LOAD_IMAGE_GRAYSCALE
: If set to this constant, always converts the image to grayscale
To save images, we can use the imwrite
function, which stores a matrix image in our computer:
// Write images imwrite("lenaGray.jpg", gray);
The first parameter is the path where we want to save the image with the extension format that we desire. The second parameter is the matrix image that we want to save. In our code sample, we create and store a gray version of the image and then save it as a jpg file the gray image that we loaded and store in gray
variable:
// Get same pixel with opencv function int myRow=color.cols-1; int myCol=color.rows-1;
Using the .cols
and .rows
attributes of a matrix, we can access the number of columns and rows of an image—or in other words, the width and height:
Vec3b pixel= color.at<Vec3b>(myRow, myCol); cout << "Pixel value (B,G,R): (" << (int)pixel[0] << "," << (int)pixel[1] << "," << (int)pixel[2] << ")" << endl;
To access one pixel of an image, we use the cv::Mat::at<typename t>(row,col)
template function from the Mat
OpenCV class. The template parameter is the desired return type. A typename
in an 8-bit color image is a Vec3b
class that stores three unsigned char data (Vec=vector, 3=number of components, and b = 1 byte). In the case of the gray image, we can directly use the unsigned char or any other number format used in the image, such as uchar pixel= color.at<uchar>(myRow, myCol)
:
// show images imshow("Lena BGR", color); imshow("Lena Gray", gray); // wait for any key press waitKey(0);
Finally, to show the images, we can use the imshow
function that creates a window with a title as the first parameter and the image matrix as the second parameter.
Note
If we want to stop our application by waiting for the user to press a key, we can use the OpenCV waitKey
function with a parameter set to the number of milliseconds we want to wait. If we set the parameter to 0
, then the function will wait forever.
The result of this code is shown in the following picture; the left-hand image is a color image and right-hand image is a gray scale:

Finally, as an example for the following samples, we are going to create the CMakeLists.txt
to allow you to compile our project and also see how to compile it.
The following code describes the CMakeLists.txt
file:
cmake_minimum_required (VERSION 2.6) cmake_policy(SET CMP0012 NEW) PROJECT(project) # Requires OpenCV FIND_PACKAGE( OpenCV 3.0.0 REQUIRED ) MESSAGE("OpenCV version : ${OpenCV_VERSION}") include_directories(${OpenCV_INCLUDE_DIRS}) link_directories(${OpenCV_LIB_DIR}) ADD_EXECUTABLE( sample main.cpp ) TARGET_LINK_LIBRARIES( sample ${OpenCV_LIBS} )
To compile our code, using the CMakeLists.txt
file we have to perform the following steps:
- Create a
build
folder. - Inside the
build
folder, executecmake
or open theCMake gui
app in Windows, choose the source folder and build folder, and click on the Configure and Generate buttons. - After step 2, if we are in Linux or OS, generate a
makefile
; then we have to compile the project using the make command. If we are in Windows, we have to open the project with the editor that we selected in step 2 and compile it. - After step 3, we have an executable called
app
.