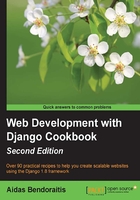
Using model mixins
In object-oriented languages, such as Python, a mixin class can be viewed as an interface with implemented features. When a model extends a mixin, it implements the interface and includes all its fields, properties, and methods. Mixins in Django models can be used when you want to reuse the generic functionalities in different models multiple times.
Getting ready
First, you will need to create reusable mixins. Some typical examples of mixins are given later in this chapter. A good place to keep your model mixins is in the utils
module.
Tip
If you create a reusable app that you will share with others, keep the model mixins in the reusable app, for example, in the base.py
file.
How to do it…
Open the models.py
file of any Django app, where you want to use the mixins and type the following code:
# demo_app/models.py
# -*- coding: UTF-8 -*-
from __future__ import unicode_literals
from django.db import models
from django.utils.translation import ugettext_lazy as _
from django.utils.encoding import python_2_unicode_compatible
from utils.models import UrlMixin
from utils.models import CreationModificationMixin
from utils.models import MetaTagsMixin
@python_2_unicode_compatible
class Idea(UrlMixin, CreationModificationMixin, MetaTagsMixin):
title = models.CharField(_("Title"), max_length=200)
content = models.TextField(_("Content"))
class Meta:
verbose_name = _("Idea")
verbose_name_plural = _("Ideas")
def __str__(self):
return self.title
How it works…
Django model inheritance supports three types of inheritance: abstract base classes, multi-table inheritance, and proxy models. Model mixins are abstract model classes with specified fields, properties, and methods. When you create a model such as Idea
, as shown in the preceding example, it inherits all the features from UrlMixin
, CreationModificationMixin
, and MetaTagsMixin
. All the fields of the abstract classes are saved in the same database table as the fields of the extending model. In the following recipes, you will learn how to define your model mixins.
Note that we are using the @python_2_unicode_compatible
decorator for our Idea
model. As you might remember from the Making your code compatible with both Python 2.7 and Python 3 recipe in Chapter 1, Getting Started with Django 1.8, it's purpose is to make the __str__()
method compatible with Unicode for both the following Python versions: 2.7 and 3.
There's more…
To learn more about the different types of model inheritance, refer to the official Django documentation available at https://docs.djangoproject.com/en/1.8/topics/db/models/#model-inheritance.
See also
- The Making your code compatible with both Python 2.7 and Python 3 recipe in Chapter 1, Getting Started with Django 1.8
- The Creating a model mixin with URL-related methods recipe
- The Creating a model mixin to handle creation and modification dates recipe
- The Creating a model mixin to take care of meta tags recipe