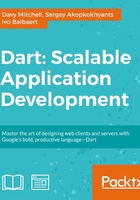
Logging
Web servers such as Apache and IIS track a visitor's web browser usage, which helps webmasters understand how visitors use their site and how many people view the pages. This good practice also helps web developers find problems in their site and helps the developers of a web server software diagnose issues.
Writing text files
With dart:io
, we already read files from the file system, and writing operates in a similar manner, as follows:
import 'dart:io'; void main() { File myfile = new File("example.txt"); myfile.writeAsStringSync("Hello Word!"); }
This demonstrates the synchronous version of the function, with writeAsString
being the asynchronous version.
Open the log.dart
file in this chapter's project. For this, take a look at the following code snippet:
void log(HttpRequest request) { String entry = getLogEntry(request); File logstore = new File("accesslog.txt"); logstore.writeAsStringSync(entry, mode: FileMode.APPEND); }
To record the web access request to the disk, the log entries will have to be accumulative so that the entries are written with the APPEND
mode passed as a named parameter.
Extracting request information
The HttpRequest
object and headers
property expose metadata from the request to the client. For example, the headers
property can give the host and port number. The user-agent identifies which program or web browser was used when making the request, as shown in the following code snippet:
String getLogEntry(HttpRequest request) { //USER_AGENT HttpHeaders headers = request.headers; String reqUri = "${headers.host},${headers.port}${request.uri.toString()}"; String entry = " ${request.connectionInfo.remoteAddress.address}, $reqUri, ${headers[HttpHeaders.USER_AGENT]}\r\n"; return (new DateTime.now()).toString() + entry; }
The remoteAddress
getter (IP address) can be used to track who was browsing and from which part of the world. Log entries to request the index.html
blog would look as follows:
2015-05-17 17:03:48.496 127.0.0.1, 127.0.0.1,8080/index.html, [Mozilla/5.0 (X11; CrOS x86_64 6812.88.0) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/42.0.2311.153 Safari/537.36] 2015-05-17 17:03:49.177 127.0.0.1, 127.0.0.1,8080/6.png, [Mozilla/5.0 (X11; CrOS x86_64 6812.88.0) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/42.0.2311.153 Safari/537.36] 2015-05-14 18:52:45.690 127.0.0.1, 127.0.0.1,8080/favicon.ico, [NetSurf/2.9 (Linux; x86_64)]
Browsers will request the page that is browsed to by the user and also other assets such as favicon.ico
(the small square graphic that is often shown in the URL bar of a web browser or on a bookmark).