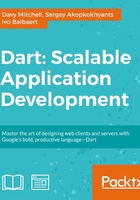
Going fullscreen
A slide show has been in the small screen mode so far and needs to be moved to a web browser equivalent of IMAX—fullscreen mode. The audience doesn't want to see a slideshow editor or desktop—just the slides.
Request fullscreen
While the trend in web browsers has been to gradually reduce the size of screen controls—a trend accelerated by the Chrome browser—fullscreen web applications are still being accepted by users and standards are settled between the browsers. Lets have a look at the following screenshot:
For security, the web browser will prompt the user to give permission for the website to enter the fullscreen mode, which hides toolbars and status bars that are offscreen.

Once the fullscreen mode is entered, the user is notified of the domain that takes over the entire screen. The web browser also sets the Escape (Esc) key as a means for the user to exit the fullscreen mode. After a few seconds, it floats off the screen and can be brought back by moving the pointer back to the top of the screen.
The exact behavior and positioning may vary according to your web browser and operating system. For example, Internet Explorer tends to display notifications at the bottom of the page.

The requestFullscreen
method is available on most page elements, though typically, div
will be used as a container. The presenter application calls the setupFullScreen
method from the constructor of the SlideShowApp
object. A Boolean flag is used to keep track of the display mode:
qs("#btnStartShow").onClick.listen((e) { isFullScreen = true; liveFullScreen ..requestFullscreen() ..focus() ..classes.toggle("fullScreenShow") ..style.visibility = "visible"; liveSlideID = 0; updateLiveSlide(); });
The onFullscreenChange
event can be listened to so that an individual element can listen to the change in state. For example, a toolbar may not appear when the application is in fullscreen mode.
When you click on div
, the isFullScreen
flag is set, fullscreen is requested, and the input focus is set on the liveFullScreen
object. Next, the .css
file is set on div
and it is made visible on screen. Finally, liveSlideID
is set at the start of the presentation and the display is updated with the slide's contents.
Updating the interface for fullscreen
The editor screen will need a button to start the slideshow in fullscreen mode. The display will be an entirely new element and not just an enlarged editor preview.
Fullscreen will be a div
element that fills the entire window and contains child div
elements for background, complete with a background image, and the slide that is currently being displayed.
Updating keyboard controls for fullscreen
An event listener similar to the one implemented for the editor screen in the previous chapter is required here. The keyboard controls are set up in the setupKeyboardNavigation
method of the SlideShowApp
class:
void setupKeyboardNavigation() { //Keyboard navigation. window.onKeyUp.listen((KeyboardEvent e) { if (isFullScreen) { if (e.keyCode == 39) { liveSlideID++; updateLiveSlide(); } else if (e.keyCode == 37) { liveSlideID--; updateLiveSlide(); } } else { //Check the editor does not have focus. if (presEditor != document.activeElement) { if (e.keyCode == 39) showNextSlide(); else if (e.keyCode == 37) showPrevSlide(); else if (e.keyCode == 38) showFirstSlide(); else if (e.keyCode == 40) showLastSlide(); } } });
The isFullScreen
flag is used to determine which mode the presentation application is in. When in the fullscreen mode, liveSlideID
is incremented or decremented depending on the key pressed and the currently updated displayed slide.
Clicking on the mouse button to advance the presentation is a fairly common feature and our application should support this too. This is implemented in the setupFullScreen
method:
liveFullScreen = qs("#presentationSlideshow"); liveFullScreen.onClick.listen((e) { liveSlideID++; updateLiveSlide(); });
The onClick
event is listened to on the fullscreen div
element, and the slideshow is advanced to the next slide when this request is received. Let's have a look at the following screenshot:
