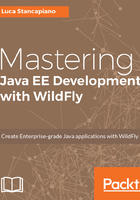
上QQ阅读APP看书,第一时间看更新
The BeanManager object
The BeanManager is the interface to the CDI engine. It can be injected in any bean as follows:
@Inject
BeanManager beanManager
Through it, you can programmatically operate the managed beans. For example, you can do an injection or a disposal of the bean, or you can annotate it at runtime and declare it. Here is a sample of manual injection:
Set<Bean<?>> beans = beanManager.getBeans(CommonBean.class);
assertEquals("One injected common bean", 1, beans.size());
@SuppressWarnings("unchecked")
Bean<CommonBean> bean = (Bean<CommonBean>) beanManager.resolve(beans);
CommonBean cb = beanManager.getContext(bean.getScope()).get(bean, beanManager.createCreationalContext(bean));
final String greeting = cb.sayHello(user);
It can be invoked manually through Java Naming and Directory Interface (JNDI) too:
BeanManager bm = null;
try {
InitialContext context = new InitialContext();
bm = (BeanManager) context.lookup("java:comp/BeanManager");
} catch (Exception e) {
e.printStackTrace();
}
Or through the @Resource annotation:
@Resource(lookup = "java:comp/BeanManager")
private BeanManager beanManager;
Java EE 7 introduces two new service provider interfaces for the CDI engine with the same features of the BeanManager, but they can be used statically without being injected.