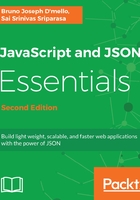
JSON implementation in PHP
PHP is considered to be one of the most popular languages for building web applications. It is a server-side scripting language and allows developers to build applications that can perform operations on the server, connect to a database to perform CRUD (Create, Read, Update, Delete) operations, and provide a stately environment for real-time applications. JSON support has been built into the PHP core from PHP 5.2.0; this helps users avoid going through any complex installations or configurations. Given that JSON is just a data interchange format, PHP consists of two functions. These functions handle JSON that comes in via a request or generate JSON that will be sent via a response. PHP is a weakly-typed language; for this example, we will use the data stored in a PHP array and convert that data into JSON string, which can be utilized as a data feed. Let us recreate the student example that we used in an earlier section, build it in PHP, and convert it into JSON:
<?php
$student = array(
"id"=>101,
"name"=>"John Doe",
"isStudent"=>true,
"scores"=>array(40, 50);
"courses"=>array(
"major"=>"Finance",
"minor"=>"Marketing"
);
);
//Echo is used to print the data
echo json_encode($student); //encoding the array into JSON string
?>
This script starts by initializing a variable and assigning an associative array that contains student information. The variable $students is then passed to a function called json_encode(), which converts the variable into JSON string. When this script is run, it generates a valid response that can be exposed as JSON data feed for other applications to utilize.
The output is as follows:
{
"id": 101,
"name": "John Doe",
"isStudent": true,
"scores": [40, 50],
"courses":
{
"major": "Finance",
"minor": "Marketing"
}
}
We have successfully generated our first JSON feed via a simple PHP script; let us take a look at the method to parse JSON that comes in via an HTTP request. It is common for web applications that make asynchronous HTTP requests to send data in JSON format:
$student = '{"id":101,"name":"John Doe","isStudent":true,"scores":[40,50],"courses":{"major":"Finance","minor":"Marketing"}}';
//Decoding JSON string into php array
print_r(json_decode($student));
The output is as follows:
Object(
[id] => 101
[name] => John Doe
[isStudent] => 1
[scores] => Array([0] => 40[1] => 50)
[courses] => stdClass
Object([major] => Finance[minor] => Marketing)
)