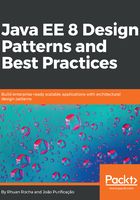
Implementing DownloadApplicationController
Here, we have the implementation of DownloadApplicationController, which is responsible for deciding the correct command to send the request. The process for deciding the correct command can be carried out in several ways, with reflections and annotations, using switch cases and maps, among others. In our example, we use a map to help us:
import com.rhuan.action.Command.AbstractCommand;
import com.rhuan.action.Command.PdfCommand;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
public class DownloadApplicationController {
private static Logger logger = LogManager.getLogger(DownloadApplicationController.class);
private final String PAGE_ERROR = "/pageError.jsp";
private HttpServletRequest request;
private HttpServletResponse response;
private Map<String, Class> map;
private String key;
public DownloadApplicationController(HttpServletRequest
request, HttpServletResponse
response){
//On our example, only PDF and JPG is acepted to download.
this.map = new HashMap<String, Class>();
this.map.put("PDF", PdfCommand.class);
this.map.put("JPG", PdfCommand.class);
this.request = request;
this.response = response;
}
public void process(){
//Processes the URI and creates the key using URI.
this.processUri();
//Validates if the request is valid.
if (!validate()) {
try {
response.sendError(400);
} catch (IOException e1) {
logger.error(e1.getMessage());
}
return;
}
//Get the correspondent command.
Class commandClass = map.get(key);
boolean error = false;
try {
AbstractCommand command = (AbstractCommand)
commandClass.newInstance();
//Executes the command.
command.execute(request,response);
} catch (InstantiationException e) {
logger.error(e.getMessage());
error = true;
} catch (IllegalAccessException e) {
logger.error(e.getMessage());
error = true;
} catch (ServletException e) {
logger.error(e.getMessage());
error = true;
} catch (IOException e) {
logger.error(e.getMessage());
error = true;
}
//If an error ocorred, response 500.
if(error){
try {
response.sendError(500);
} catch (IOException e1) {
logger.error(e1.getMessage());
return;
}
}
}
private void processUri(){
String uri = request.getRequestURI();
if(uri.startsWith("/")) uri = uri.replaceFirst("/", "");
String[] uriSplitted = uri.split("/");
if(uriSplitted.length > 2)
key = uriSplitted[2].toUpperCase();
}
private boolean validate(){
String uri = request.getRequestURI();
if(uri.startsWith("/")) uri = uri.replaceFirst("/", "");
String[] uriSplitted = uri.split("/");
return uriSplitted.length == 3 && map.containsKey(key);
}
}
In the preceding code, we created two methods to help us to process and validate the URI, the processUri method, and the validated method. After processing the URI, validating the request and generating the key, the code finds the correct command using the key generated. In the following code snippet, we have the line of code that gets the command class by key, creates a new instance of the command, and executes the following command:
//Get the correspondent command.
Class commandClass = map.get(key);
A new instance of the command is then created using the newInstance() method :
//Instantiate the command
AbstractCommand command = (AbstractCommand) commandClass.newInstance();
The command is then executed, passing request and response as parameters:
//Executes the command.
command.execute(request,response);