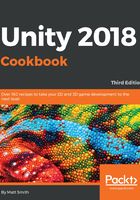
上QQ阅读APP看书,第一时间看更新
How to do it...
To display text to inform the user about the status of carrying a single object pickup, follow these steps:
- Start with a new copy of the Simple2Dgame_SpaceGirl mini-game.
- Add a UI Text object (Create | UI | Text). Rename it Text-carrying-star. Change its text to Carrying star: false.
- Import the provided Fonts folder into your project.
- In the Inspector panel, set the font of Text-carrying-star to Xolonium-Bold, and set its color to yellow. Center the text horizontally and vertically, set its Height to 50, and set the Font Size to 32.
- Edit its Rect Transform, and while holding down Shift + Alt (to set pivot and position), choose the top-stretch box:

- Your text should now be positioned at the middle top of the Game panel, and its width should stretch to match that of the whole panel, as shown in the screenshot in the introduction to this recipe.
- Create the following C# script class PlayerInventory in the _Scripts folder:
using UnityEngine; public class PlayerInventory : MonoBehaviour { private PlayerInventoryDisplay playerInventoryDisplay; private bool carryingStar = false; void Awake() { playerInventoryDisplay = GetComponent<PlayerInventoryDisplay>(); } void Start() { playerInventoryDisplay.OnChangeCarryingStar( carryingStar); } void OnTriggerEnter2D(Collider2D hit) { if (hit.CompareTag("Star")) { carryingStar = true; playerInventoryDisplay.OnChangeCarryingStar( carryingStar); Destroy(hit.gameObject); } } }
- Create the following C# script class PlayerInventoryDisplay in the _Scripts folder:
using UnityEngine; using UnityEngine.UI; [RequireComponent(typeof(PlayerInventory))] public class PlayerInventoryDisplay : MonoBehaviour { public Text starText; public void OnChangeCarryingStar(bool carryingStar) { string starMessage = "no star :-("; if(carryingStar) starMessage = "Carrying star :-)"; starText.text = starMessage; } }
- Add an instance of script-class PlayerInventoryDisplay to the player-SpaceGirl GameObject in the Hierarchy.
Note, since the PlayerInventoryDisplay class contains RequireComponent(), then an instance of script class PlayerInventory will be automatically added to GameObject player-SpaceGirl.
- From the Hierarchy view, select the player-SpaceGirl GameObject. Then, from the Inspector, access the Player Inventory Display (Script) component and populate the Star Text public field with GameObject Text-carrying-star, as shown in the screenshot.

- When you play the scene, after moving the character into the star, the star should disappear, and the onscreen UI Text message should change to Carrying star :-):
