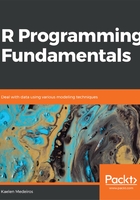
While loop
Versus the for loop, which walks through an iterator (usually, this is a sequence of numbers), a while loop will not iterate through a sequence of numbers for you. Instead, it requires you to add a line of code inside the body of the loop that increments or decrements your iterator, usually i. Generally, the syntax for a while loop is as follows:
while(test_expression){
some_action
}
Here, the action will only occur if the test_expression is TRUE. Otherwise, R will not enter the curly braces and run what's inside them. If a test expression is never TRUE, it's possible that a while loop may never run!
A classic example of a while loop is one that prints out numbers, such as the following:
i = 0
while(i <= 5){
print(paste("loop", i))
i = i + 1
}
The output of the preceding code is as follows:

Because we set our test expression to be i less than or equal to 5, the loop stopped printing once i was 6, and R broke out of the while loop. This is good, because infinite loops (loops that never stop running) are definitely possible. If the while loop test expression is never FALSE, the loop will never stop, as shown in the following code:
while(TRUE){
print("yes!")
}
The output will be as follows:
[1] "yes!"
[1] "yes!"
[1] "yes!"
[1] "yes!"
……
This is an example of an infinite loop. If you're concerned about them, R does have a break statement, which will jump out of the while loop, but you'll see the following error:
Error: no loop for break/next, jumping to top level
This is because break statements in R are meant more for breaking out of nested loops, where there is one inside another.
It's also possible (though you likely wouldn't code this on purpose, as it will be an error of some kind) for a while loop to never run. For example, if we forgot that i is in our global environment, and that it equals 5, the following loop will never run:
while(i < 5){
print(paste(i, "is this number"))
i = i + 1
}
Let's now try and get a feel of how loops work in R. We will try to predict what the loop code will print. Follow the steps below:
- Examine the following code snippet. Try to predict what the output will be:
vec <- seq(1:10)
for(num in seq_along(vec)){
if(num %% 2 == 0){
print(paste(num, "is even"))
} else{
print(paste(num, "is odd"))
}
}
- Examine the following code snippet. Try to predict what the output will be:
example <- data.frame(color = c("red", "blue", "green"), number = c(1, 2, 3))
for(i in seq(nrow(example))){
print(example[i,1])
}
- Examine the following code snippet. Try to predict what the output will be:
var <- 5
while(var > 0){
print(var)
var = var - 1
}
Output: The output for the first step will be as follows:

The output for the second step will be as follows:

The output for the third step will be as follows:

It's important as you code in R that you understand how loops work, both because other people will write code with them and so you need to understand how other methods that can be substituted in for loops in R work.