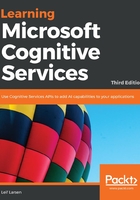
Identifying a person
To identify a person, we are first going to upload an image. Open the HomeView.xaml
file and add a ListBox
element to the UI. This will contain the person groups to choose from when identifying a person. We will need to add a button element to find an image, upload it, and identify the person. A TextBox
element is added to show the working response. For our own convenience, we also add an image element to show the image we are using.
In the View
model, add an ObservableCollection
property of a PersonGroup
type. We need to add a property for the selected PersonGroup
type. Also, add a BitmapImage
property for our image, and a string property for the response. We will also need an ICommand
property for our button.
Add a private
variable for the FaceServiceClient
type, as follows:
private FaceServiceClient _faceServiceClient;
This will be assigned in our constructor, which should accept a parameter of a FaceServiceClient
type. From the constructor, call on the Initialize
function to initialize everything, as shown in the following code:
private void Initialize() { GetPersonGroups(); UploadOwnerImageCommand = new DelegateCommand(UploadOwnerImage,CanUploadOwnerImage); }
First, we call the GetPersonGroups
function to retrieve all the person groups. This function makes a call to the ListPersonGroupsAsync
API, which we saw earlier. The result is added to our PersonGroup
list's ObservableCollection
parameter.
Next, we create our ICommand
object. The CanUploadOwnerImage
function will return true
if we have selected an item from the PersonGroup
list. If we have not, it will return false
, and we will not be able to identify anyone.
In the UploadOwnerImage
function, we first browse to an image and then load it. With an image loaded and a file path available, we can start to identify the person in the image, as shown in the following code:
using (StreamimageFile = File.OpenRead(filePath)) { Face[] faces = await _faceServiceClient.DetectAsync(imageFile); Guid[] faceIds = faces.Select(face =>face.FaceId).ToArray();
We open the image as a Stream
type, as shown in the following code. Using this, we detect faces in the image. From the detected faces, we get all the face IDs in an array:
IdentifyResult[] personsIdentified = await _faceServiceClient.IdentifyAsync (SelectedPersonGroup.PersonGroupId, faceIds, 1);
The array of face IDs will be sent as the second parameter to the IdentifyAsync
API call. Remember that when we detect a face, it is stored for 24 hours. Proceeding to use the corresponding face ID will make sure that the service knows which face to use for identification.
The first parameter used is the ID of the person group we have selected. The last parameter in the call is the number of candidates returned. As we do not want to identify more than one person at a time, we specify one. Because of this, we should ensure that there is only one face in the image we upload.
A successful API call will result in an array of the IdentifyResult
parameter, as shown in the following code. Each item in this array will contain candidates:
foreach(IdentifyResultpersonIdentified in personsIdentified) { if(personIdentified.Candidates.Length == 0) { SystemResponse = "Failed to identify you."; break; } GuidpersonId = personIdentified.Candidates[0].PersonId;
We loop through the array of results, as shown in the following code. If we do not have any candidates, we just break out of the loop. If, however, we do have candidates, we get the PersonId
parameter of the first candidate (we asked for only one candidate earlier, so this is okay):
Person person = await faceServiceClient.GetPersonAsync( SelectedPersonGroup.PersonGroupId, personId); if(person != null) { SystemResponse = $"Welcome home, {person.Name}"; break; } } }
With the personId
parameter, we get a single Person
object, using the API to call the GetPersonAsync
function. If the call is successful, we print a welcome message to the correct person (as shown in the following screenshot) and break out of the loop:
