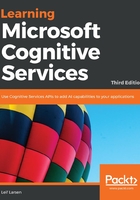
Setting up a chapter example project
Before we go into the specifics of the API, we need to create an example project for this chapter. This project will contain all of the examples, which will not be put into the end-to-end application at this stage:
Note
If you have not already done so, sign up for an API key for Computer Vision by visiting https://portal.azure.com.
- Create a new project in Visual Studio using the template we created in Chapter 1, Getting Started with Microsoft Cognitive Services.
- Right-click on the project and choose Manage NuGet Packages. Search for the
Microsoft.ProjectOxford.Vision
package and install it into the project, as shown in the following screenshot: - Create the following
UserControls
files and add them into theViewModel
folder:CelebrityView.xaml
DescriptionView.xaml
ImageAnalysisView.xaml
OcrView.xaml
ThumbnailView.xaml
- Also, add the corresponding
ViewModel
instances from the following list into theViewModel
folder:CelebrityViewModel.cs
DescriptionViewModel.cs
ImageAnalysisViewModel.cs
OcrViewModel.cs
ThumbnailViewModel.cs
Go through the newly created ViewModel
instances and make sure that all classes are public.
We will switch between the different views using a TabControl
tag. Open the MainView.xaml
file and add the following in the precreated Grid
tag:
<TabControl x: Name = "tabControl" HorizontalAlignment = "Left" VerticalAlignment = "Top" Width = "810" Height = "520"> <TabItem Header="Analysis" Width="100"> <controls:ImageAnalysisView /> </TabItem> <TabItem Header="Description" Width="100"> <controls:DescriptionView /> </TabItem> <TabItem Header="Celebs" Width="100"> <controls:CelebrityView /> </TabItem> <TabItem Header="OCR" Width="100"> <controls:OcrView /> </TabItem> <TabItem Header="Thumbnail" Width="100"> <controls:ThumbnailView /> </TabItem> </TabControl>
This will add a tab bar at the top of the application that will allow you to navigate between the different views.
Next, we will add the properties and members required in our MainViewModel.cs
file.
The following is the variable used to access the Computer Vision API:
private IVisionServiceClient _visionClient;
The following code declares a private variable holding the CelebrityViewModel
object. It also declares the public
property that we use to access the ViewModel
in our View
:
private CelebrityViewModel _celebrityVm; public CelebrityViewModel CelebrityVm { get { return _celebrityVm; } set { _celebrityVm = value; RaisePropertyChangedEvent("CelebrityVm"); } }
Following the same pattern, add properties for the rest of the created ViewModel
instances.
With all the properties in place, create the ViewModel
instances in our constructor using the following code:
public MainViewModel() { _visionClient = new VisionServiceClient("VISION_API_KEY_HERE", "ROOT_URI"); CelebrityVm = new CelebrityViewModel(_visionClient); DescriptionVm = new DescriptionViewModel(_visionClient); ImageAnalysisVm= new ImageAnalysisViewModel(_visionClient); OcrVm = new OcrViewModel(_visionClient); ThumbnailVm = new ThumbnailViewModel(_visionClient); }
Note how we first create the VisionServiceClient
object with the API key that we signed up for earlier and the root URI, as described in Chapter 1, Getting Started with Microsoft Cognitive Services. This is then injected into all the ViewModel
instances to be used there.
This should now compile and present you with the application shown in the following screenshot:
