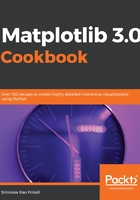
上QQ阅读APP看书,第一时间看更新
There's more...
Let's take one more example of how to customize the size, length, and color of ticks. The following code block displays three plots; the first one uses default settings for ticks and ticklabels, and the second one is customized to display major and minor ticks, and the third one is customized to specify the length, thickness, and color of the major and minor ticks:
- Define the function for formatting numeric data:
def Num_Format(x, pos):
"""The two arguments are the number and tick position"""
if x >= 1e6:
string = '${:1.1f}M'.format(x*1e-6)
else:
string = '${:1.0f}K'.format(x*1e-3)
return string
- Define a function to plot the graph:
def plot_graph(axes, axis, major_step_size, minor_step_size):
majorLocator = MultipleLocator(major_step_size)
minorLocator = MultipleLocator(minor_step_size)
if axis == 'x':
axes.xaxis.set_major_locator(majorLocator)
axes.xaxis.set_minor_locator(minorLocator)
else:
axes.yaxis.set_major_locator(majorLocator)
axes.yaxis.set_minor_locator(minorLocator)
axes.yaxis.set_major_formatter(formatter)
- Set up the data for the plot:
x = np.linspace(-50,50,500)
y = x**2 + np.cos(x)*100
- Define the figure, layout, and size with adjustment for space in between the plots:
fig, axs = plt.subplots(1,3, figsize=(15,5))
fig.subplots_adjust(wspace=0.5)
- Plot the graph with the formatter applied to the y axis:
formatter = FuncFormatter(Num_Format)
axs[0].plot(x, y**2)
axs[0].set_title('Default Ticks and Ticklabels')
axs[0].yaxis.set_major_formatter(formatter)
- Plot the line graph on the second axis with major and minor ticks:
axs[1].plot(x, y**2)
plot_graph(axs[1], 'y', 500000, 250000)
plot_graph(axs[1], 'x', 10, 2)
axs[1].set_title('Major and Minor Ticks')
- Plot the line graph on axis three with customized ticks and ticklabels:
axs[2].plot(x, y**2)
plot_graph(axs[2], 'x', 10, 2)
minorLocator = AutoMinorLocator()
axs[2].xaxis.set_minor_locator(minorLocator)
axs[2].tick_params(which='major', length=10, color='g')
axs[2].tick_params(which='minor', length=4, color='r')
axs[2].tick_params(which='both', width=2)
axs[2].set_title('Customised Ticks')
axs[2].grid(True)
- Display the figure on the screen:
plt.show()
Here is the explanation of the code and how it works:
- The first plot on axis zero, uses default settings, and it automatically chooses one million bins for the y axis, and 20 unit bins for the x axis, and places ticks and ticklabels accordingly.
- The second plot on axis one, majorLocator = MultipleLocator(500000), specifies that major ticks should be placed at 500000 intervals, and axs[1].yaxis.set_major_locator(majorLocator) sets this interval on the y axis major tick. majorFormatter = FormatStrFormatter('%d') specifies the format of the ticklabel number to be displayed, and axs[1].yaxis.set_major_formatter(majorFormatter) applies the format to the y axis major ticklabel.
- The same process is repeated for the x axis with 10 unit bins for major ticks and 2 units for minor ticks.
- The third plot is similar to the second one, limiting minor ticks only to the x axis, and the addition of setting length, width, and color for both major and minor ticks. tick_params() sets these parameters, with the argument which= specifying major, minor, or both ticks to be applied.
Here is the generated output for the preceding code: