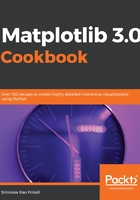
上QQ阅读APP看书,第一时间看更新
How to do it...
The following code block draws three plots with various labels to demonstrate how these can be customized:
- Define the figure, layout, and size, with adequate space in between the plots:
fig, ax = plt.subplots(1, 3, figsize=(10,4))
fig.subplots_adjust(wspace=0.7)
- Plot a histogram on the first axis with customized labels and title:
ax[0].hist(np.random.randn(1000))
ax[0].set(xlabel='Bins', ylabel='Frequency')
atitle = ax[0].set_title('Histogram', fontstyle='italic',
fontsize=14)
plt.setp(atitle,color='blue')
- Plot a bar graph on the second axis:
# Plot the bars for men's data
menMue = [3, 10, 100, 500, 50]
menSigma = [0.75, 2.5, 25, 125, 12.5]
index = np.arange(len(menMue)) # the x locations for the groups
width = 0.35 # the width of the bars
p1 = ax[1].bar(index, menMue, width, color='r', bottom=0,
yerr=menSigma)
# Plot the bars for women's data
womenMue = [1000, 3, 30, 800, 1]
womenSigma = [500, 0.75, 8, 200, 0.25]
p2 = ax[1].bar(index + width, womenMue, width, color='y', bottom=0,
yerr=womenSigma)
# customize title and labels for the figure
atitle = ax[1].set_title('Scores by category and gender',
fontstyle='italic', fontsize=14)
plt.setp(atitle,color='blue')
ax[1].set(xticks=(index + width / 2), xticklabels=('C1', 'C2', 'C3',
'C4', 'C5'), yscale='log')
ax[1].legend((p1[0], p2[0]), ('Men', 'Women'), bbox_to_anchor=
(1.05,1))
- Plot a scatter plot on the third axis:
ax[2].scatter(np.random.rand(100),np.random.rand(100),
s=100*np.random.rand(100)*np.random.rand(100))
atitle = ax[2].set_title('Scatter Plot', fontstyle='italic',
fontsize=14)
plt.setp(atitle,color='blue')
- Set the title for the figure:
ftitle= plt.suptitle('Figure Title', fontname='arial', fontsize=20,
fontweight='bold')
plt.setp(ftitle,color='green')
- Adjust the space between the plots and display the figure on the screen:
plt.tight_layout(pad=3, w_pad=5)
plt.show()