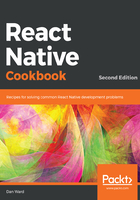
上QQ阅读APP看书,第一时间看更新
How to do it...
- In the App.js file, we're going to create a stateless component. This component will mimic a small music player. It will only display the name of the song and a bar to show the progress. The first step is importing our dependencies:
import React from 'react'; import { StyleSheet, Text, View } from 'react-native';
- Once we've imported the dependencies, we can build out the component:
export default class App extends React.Component { render() { const name = '01 - Blue Behind Green Bloches'; return ( <View style={styles.container}> <View style={styles.innerContainer} /> <Text style={styles.title}> <Text style={styles.subtitle}>Playing:</Text> {name} </Text> </View> ); } }
- We have our component ready, so now we need to add some styles, to add colors and fonts:
const styles = StyleSheet.create({ container: { margin: 10, marginTop: 100, backgroundColor: '#e67e22', borderRadius: 5, }, innerContainer: { backgroundColor: '#d35400', height: 50, width: 150, borderTopLeftRadius: 5, borderBottomLeftRadius: 5, }, title: { fontSize: 18, fontWeight: '200', color: '#fff', position: 'absolute', backgroundColor: 'transparent', top: 12, left: 10, }, subtitle: { fontWeight: 'bold', }, });
- As long as our simulator and emulator are running our application, we should see the changes:
