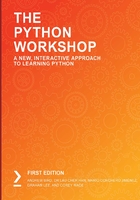
Basic Functions
A function is a reusable piece of code that is only run when it is called. Functions can have inputs, and they usually return an output. For example, using a Python shell, you can define the following function that takes two inputs and returns the sum:
def add_up(x, y):
return x + y
add_up(1, 3)
You should get the following output:
4
Exercise 42: Defining and Calling the Function in Shell
In this exercise, you create a function that will return the second element of a list if it exists:
- In a Python shell, enter the function definition. Note that the tab spacing needs to match the following output:
def get_second_element(mylist):
if len(mylist) > 1:
return mylist[1]
else:
return 'List was too small'
Here you are calling print logs the parsed message to the standard output.
- Try running the function on a small list of integers:
get_second_element([1, 2, 3])
You should get the following output:
2
- Try running the function on a list with only one element:
get_second_element([1])
You should get the following output:

Figure 3.17: We are unable to get the second item with a list length of 1
Defining functions in a shell can be difficult, as the shell isn't optimized for editing multiple lines of code blocks. Instead, it's preferable for our functions to live inside a Python script.
Exercise 43: Defining and Calling the Function in Python Script
In this exercise, you will define and call a function in a multiply.py Python script and execute it from Command Prompt:
- Create a new file using a text editor called multiply.py:
def list_product(my_list):
result = 1
for number in my_list:
result = result * number
return result
print(list_product([2, 3]))
print(list_product([2, 10, 15]))
- Using Command Prompt, execute this script, ensuring that your Command Prompt is in the same folder as the multiply.py file:

Figure 3.18: Running from the command line
In this exercise, you worked on defining and calling a function within a Python script.
Exercise 44: Importing and Calling the Function from the Shell
In this exercise, you will import and call the list_product function you defined in multiply.py:
- In a Python shell, import our list_product function:
from multiply import list_product
You should get the following output:
6
300
One unintended consequence is that your print statements in multiply.py were also executed: introduce __name__ == 'main'.
- Call the function with a new list of numbers:
list_product([-1, 2, 3])
You should get the following output:
–6
Now that you've completed this exercise, you have gained an understanding of how to import and call a function. You created the multiply.py file in Exercise 43, Defining and Calling the Function in Python Script, and imported and used this function in this exercise.
Positional Arguments
The preceding examples have all included positional arguments. In the following example, there are two positional arguments, x and y, respectively. When you call this function, the first value you pass in will be assigned to x, and the second value will be assigned to y:
def add_up(x, y):
return x + y
You can also specify functions without any arguments:
from datetime import datetime
def get_the_time():
return datetime.now()
print(get_the_time())
You should get the following output:

Figure 3.19: The current date and time
Keyword Arguments
Keyword arguments, also known as named arguments, are optional inputs to functions. These arguments have a default value that is taken when the function is called without the keyword argument specified.
Exercise 45: Defining the Function with Keyword Arguments
In this exercise, you will use the Python shell to define an add_suffix function that takes an optional keyword argument:
- In a Python shell, define the add_suffix function:
def add_suffix(suffix='.com'):
return 'google' + suffix
- Call the add_suffix function without specifying the suffix argument:
add_suffix()
You should get the following output:
'google.com'
- Call the function with a specific suffix argument:
add_suffix('.co.uk')
You should get the following output:
'google.co.uk'
Exercise 46: Defining the Function with Positional and Keyword Arguments
In this exercise, you use the Python shell to define a convert_usd_to_aud function that takes a positional argument and an optional keyword argument:
- In a Python shell, define the convert_usd_to_aud function:
def convert_usd_to_aud(amount, rate=0.75):
return amount / rate
- Call the convert_usd_to_aud function without specifying the exchange rate argument:
convert_usd_to_aud(100)
You should get the following output:
133.33333333333334
- Call the convert_usd_to_aud function with a specific exchange rate argument:
convert_usd_to_aud(100, rate=0.78)
You should get the following output:
128.2051282051282
The rule of thumb is to simply use positional arguments for required inputs that must be provided each time the function is called, and keyword arguments for optional inputs.
You will sometimes see functions that accept a mysterious-looking argument: **kwargs. This allows the function to accept any keyword arguments when it's called, and these can be accessed in a dictionary called "kwargs". Typically, this is used when you want to pass arguments through to another function.
Exercise 47: Using **kwargs
In this exercise, you will write a Python script to pass named arguments through a convert_usd_to_aud function:
- Using a text editor, create a file called conversion.py.
- Enter the convert_usd_to_aud function defined in the previous exercise:
def convert_usd_to_aud(amount, rate=0.75):
return amount / rate
- Create a new convert_and_sum_list function that will take a list of amounts, convert them to AUD, and return the sum:
def convert_and_sum_list(usd_list, rate=0.75):
total = 0
for amount in usd_list:
total += convert_usd_to_aud(amount, rate=rate)
return total
print(convert_and_sum_list([1, 3]))
- Execute this script from Command Prompt:
Figure 3.20: Converting a list of USD amounts to AUD
Note that the convert_and_sum_list function didn't need the rate argument. It simply needed to pass it through to the convert_usd_to_aud function. Imagine that, instead of one argument, you had 10 that needed to be passed through. There will be a lot of unnecessary code. Instead, you will use the kwargs dictionary.
- Add the following function to conversion.py:
def convert_and_sum_list_kwargs(usd_list, **kwargs):
total = 0
for amount in usd_list:
total += convert_usd_to_aud(amount, **kwargs)
return total
print(convert_and_sum_list_kwargs([1, 3], rate=0.8))
- Execute this script from Command Prompt:

Figure 3.21: Altering the result by specifying the kwarg rate
Activity 9: Formatting Customer Names
Suppose that you are building a Customer Relationship Management (CRM) system, and you want to display a user record in the following format: John Smith (California). However, if you don't have a location in your system, you just want to see "John Smith."
Create a format_customer function that takes two required positional arguments, first_name and last_name, and one optional keyword argument, location. It should return a string in the required format.
The steps are as follows:
- Create the customer.py file.
- Define the format_customer function.
- Open a Python shell and import your format_customer function.
- Try running a few examples. The outputs should look like this:
from customer import format_customer
format_customer('John', 'Smith', location='California')
You should get the following output:

Figure 3.22: The formatted customer name
format_customer('Mareike', 'Schmidt')
You should get the following output:

Figure 3.23: Omitting the location
Note
The solution for this activity is available on page 530.