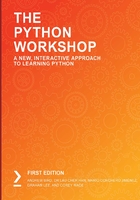
A Survey of Sets
So far, in this chapter, you have covered lists, dictionaries, and tuples. You can now have a look at sets, which are another type of Python data structure.
Sets are a relatively new addition to the Python collection type. They are unordered collections of unique and immutable objects that support operations mimicking mathematical set theory. As sets do not allow multiple occurrences of the same element, they can be used to effectively prevent duplicate values.
A set is a collection of objects (called members or elements). For instance, you can define set A as even numbers between 1 to 10, and it will contain {2,4,6,8,10}, and set B can be odd numbers between 1 to 10, and it will contain {1,3,5,7,9}. In the following exercise, you will get our hands on sets in Python:

Figure 2.21: Set A and Set B – each set contains a unique, distinct value
Exercise 32: Using Sets in Python
In this exercise, you will gain an understanding of sets in Python. A set is a collection of objects:
- Open a Jupyter notebook.
- Initialize a set using the following code. You can pass in a list to initialize a set:
s1 = set([1,2,3,4,5,6])
print(s1)
s2 = set([1,2,2,3,4,4,5,6,6])
print(s2)
s3 = set([3,4,5,6,6,6,1,1,2])
print(s3)
You should get the following output:
Figure 2.22: A set initialized using a list
You can see that the set is unique and unordered, so duplicate items and the original order are not preserved.
- Enter the following code in a new cell:
s4 = {"apple", "orange", "banana"}
print(s4)
You can also initialize a set using curly brackets directly.
You should get the following output:
{'apple', 'orange', 'banana'}
- Sets are mutable. Type the following code and see how we add a new item, pineapple, to an existing set, s4:
s4.add('pineapple')
print(s4)
You should get the following output:
{'apple', 'orange', 'pineapple', 'banana'}
In this exercise, you were introduced to sets in Python. In the next topic, you will dive in a bit deeper and understand the different set operations that Python has to offer you.
Set Operations
Sets support common operations such as unions and intersections. A union operation returns a single set that contains all the unique elements in both set A and B; an intersect operation returns a single set that contains unique elements that belong to set A and also belongs to set B at the same time:

Figure 2.23: Set A in union with Set B
The following figure represents the intersect operation:

Figure 2.24: Set A intersects with Set B
Now you should look at how to implement these set operations in Python in the following exercise:
Exercise 33: Implementing Set Operations
In this exercise, we will be implementing and working with set operations:
- Open a new Jupyter notebook.
- In a new cell, type the following code to initialize two new sets:
s5 = {1,2,3,4}
s6 = {3,4,5,6}
- Use the | operator or the union method for a union operation:
print(s5 | s6)
print(s5.union(s6))
You should get the following output:
Figure 2.25: Output with the union operator
- Now use the & operator or the intersection method for an intersection operation:
print(s5 & s6)
print(s5.intersection(s6))
You should get the following output:
Figure 2.26: Output with the & operator
- Use the – operator or the difference method to find the difference between two sets:
print(s5 - s6)
print(s5.difference(s6))
You should get the following output:
Figure 2.27: Output with the difference operator
- Now enter the <= operator or the issubset method to check if one set is a subset of another:
print(s5 <= s6)
print(s5.issubset(s6))
s7 = {1,2,3}
s8 = {1,2,3,4,5}
print(s7 <= s8)
print(s7.issubset(s8))
You should get the following output:
Figure 2.28: Output with the issubset method
The first two statements will return false because s5 is not a subset of s6. The last two statements will return True because s5 is a subset of s6. Do take note that <= operator is a test for the subset. A proper subset is the same as a general subset, except that the sets cannot be identical. You can try it out in a new cell with the following code.
- Check whether s7 is a formal subset of s8, and check whether a set can be a proper subset of itself by entering the following code:
print(s7 < s8)
s9 = {1,2,3}
s10 = {1,2,3}
print(s9 < s10)
print(s9 < s9)
You should get the following output:
Figure 2.29: Output if s7 is a formal subset of s8
We can see that s7 is a proper subset of s8 because there are other elements in s8 apart from all the elements of s7. But s9 is not a subset of s10 because they are identical. Therefore, a set is not a subset of itself.
- Now use the >= operator or the issuperset method to check whether one set is the superset of another. Try it using the following code in another cell:
print(s8 >= s7)
print(s8.issuperset(s7))
print(s8 > s7)
print(s8 > s8)
You should get the following output:
Figure 2.30: Output with the >= operator checking whether the set is a superset of another
The first three statements will return True because s8 is the superset of s7 and is also a proper superset of s7. The last statement will return false because no set can be a proper superset of itself.
Having completed this exercise, you now know that Python sets are useful for efficiently preventing duplicate values and are suitable for common math operations such as unions and intersections.
Note
After all the topics covered so far, you may think that sets are similar to lists or dictionaries. However, sets are unordered and do not map keys to values, so they are neither a sequence or a mapping type; they are a type by themselves.