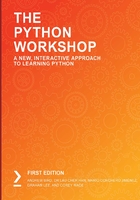
Dictionary Methods
Now that you have learned about dictionaries and when you should use a dictionary. You will now look at a few other dictionary methods. To start with, you should follow the exercises from here onward to learn how to access the values and other related operations of a dictionary in Python.
Exercise 30: Accessing a Dictionary Using Dictionary Methods
In this exercise, we will learn how to access a dictionary using dictionary methods. The goal of the exercise is to print the order values against the item while accessing the dictionary by using dictionary methods:
- Open a new Jupyter Notebook.
- Enter the following code in a new cell:
orders = {'apple':5, 'orange':3, 'banana':2}
print(orders.values())
print(list(orders.values()))
You should get the following output:
dict_values([5, 3, 2])
[5, 3, 2]
The values() method in this code returns an iterable object. In order to use the values straight away, you can wrap them in a list directly.
- Now, obtain a list of keys in a dictionary by using the keys() method:
print(list(orders.keys()))
You should get the following output:
['apple', 'orange', 'banana']
- As you can't directly iterate a dictionary, you first convert it to a list of tuples using the items() method, then iterate the resulting list and access it. This is mentioned in the following code snippet:
for tuple in list(orders.items()):
print(tuple)
You should get the following output:
('apple', 5)
('orange', 3)
('banana', 2)
In this exercise, you created a dictionary. In addition to this, you were able to list the keys mentioned in the dictionary, and later, in step 4, you were able to iterate the dictionary after converting the list to a tuple.