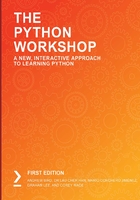
List Methods
As discussed before, since a list is a type of sequence, it supports all sequence operations and methods.
Lists are one of the best data structures to use. Python provides a set of list methods that makes it easy for us to store and retrieve values in order to maintain, update, and extract data. These common operations are what Python programmers perform, including slicing, sorting, appending, searching, inserting, and removing data.
The best way to understand this is to see them at work. You will learn about these handy list methods in the following exercises.
Exercise 25: Basic List Operations
In this exercise, you are going to use the basic functions of lists to check the size of a list, combining lists and duplicating lists as well. Follow these steps:
- Open a new Jupyter notebook.
- Type the following code
shopping = ["bread","milk", "eggs"]
- The length of a list is found using the len function.
print(len(shopping))
Note
The len() function returns the number of items in an object. When the object is a string, it returns the number of characters in the string.
You should get the following output:
3
- Now concatenate two lists using the + operator:
list1 = [1,2,3]
list2 = [4,5,6]
final_list = list1 + list2
print(final_list)
You should get the following output:
[1, 2, 3, 4, 5, 6]
As you can see in the output, lists also support many string operations as well, one of which is concatenation, which is joining two or more lists together.
- Now use the * operator, which can be used for repetition in a list to duplicate elements:
list3 = ['oi']
print(list3*3)
It will repeat 'oi' three times, giving us the following output:
['oi', 'oi', 'oi']
You have now concluded this exercise; the purpose of this exercise was to get you familiar with some common operations that Python programmers use to interact with lists.
Accessing an Item from a List
Just like other programming languages, in Python, you can use the indexer method to access elements in a list. You should complete the following exercise by continuing with the previous notebook.
Exercise 26: Accessing an Item from Shopping List Data
In this exercise, you will work with lists and gain an understanding of how you can access items from a list. The following steps will enable you to complete the exercise:
- Open a new Jupyter Notebook.
- Enter the following code in a new cell:
shopping = ["bread","milk", "eggs"]
print(shopping[1])
You should get the following output:
milk
As you can see, you have printed the value milk from the list shopping that has the index 1, as the list begins from 0.
- Now, access the milk element and replace it with banana:
shopping[1] = "banana"
print(shopping)
You should get the following output:
['bread', 'banana', 'eggs']
- Type the following code in a new cell and observe the output:
print(shopping[-1])
You should get the following output:
eggs
The output will print eggs – the last item.
Note
In Python, a positive index counts forward, and a negative index counts backward. You use a negative index to access an element from the back.
What you have learned so far is more of a traditional way of accessing elements. Python lists also support powerful general indexing, called slicing. It uses the : notation in the format of list[i:j], where i is the starting element, and j is the last element (non-inclusive).
- Enter the following code to try out a different type of slicing:
print(shopping[0:2])
This prints the first and second elements, producing the following output:
['bread', 'banana']
Now, to print from the beginning of the list to the third element
print(shopping[:3])
You should get the following output:
['bread', 'banana', 'eggs']
Similarly, to print from the second element of the list until the end
print(shopping[1:])
You should get the following output:
['banana', 'eggs']
Having completed this exercise, you are now able to access items from a list in different ways.
Adding an Item to a List
In the previous section and Exercise 26, Accessing an Item from Shopping List Data, you learned how to access items from a list. Lists are very powerful and are widely used in many circumstances. However, you often won't know the data your users want to store beforehand, but only after the program is running. Here, you are going to look at various methods for adding items to and inserting items into a list.
Exercise 27: Adding Items to Our Shopping List
The append method is the easiest way to add a new element to the end of a list. You will use this method in this exercise to add items to our shopping list. The following steps will enable you to complete the exercise:
- In a new cell, type the following code to add a new element, apple, to the end of the list using the append method:
shopping = ["bread","milk", "eggs"]
shopping.append("apple")
print(shopping)
You should get the following output:
['bread', 'milk', 'eggs', 'apple']
The append method is commonly used when you are building a list without knowing what the total number of elements will be. You will start with an empty list and continue to add items to build the list.
- Now create an empty list, shopping, and keep adding items one by one to this empty list:
shopping = []
shopping.append('bread')
shopping.append('milk')
shopping.append('eggs')
shopping.append('apple')
print(shopping)
You should get the following output:
['bread', 'milk', 'eggs', 'apple']
This way, you start off by initializing an empty list, and you extend the list dynamically. The end result is exactly the same as the list from the previous code. This is different from some programming languages that require array size to be fixed at the declaration stage.
- Now use the insert method to add elements to the shopping list:
shopping.insert(2, 'ham')
print(shopping)
You should get the following output:
['bread', 'milk', 'ham', 'eggs', 'apple']
As you coded in step 3, you came across another way to add an element to a list, using the insert method. The insert method requires a positional index to indicate where the new element should be placed. A positional index is a zero-based number that indicates the position in a list. You can use the following code to insert an item, ham, in the third position.
You can see that ham is inserted in the third position and shifts every other item one position to the right.
Having concluded this exercise, you are now able to add elements to our list, which is shopping. This proves to be very useful when you get data from a customer or client, allowing you to use the methods you have mentioned and add items to your list.
In the next topic, you will work with and learn about dictionary keys and values.