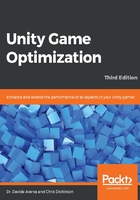
Disabling objects by distance
In other situations, we may want components or GameObjects to be disabled after they are far enough away from the player such that they may be barely visible, but too far away to matter. A good candidate for this type of activity is roaming Non-Player Character creatures: we want to see them at a distance, but where we don't need them to process anything, and therefore they can sit idle until we get closer.
The following code is a simple coroutine that periodically checks the total distance from a given target object and disables itself if it strays too far away from it:
[SerializeField] GameObject _target;
[SerializeField] float _maxDistance;
[SerializeField] int _coroutineFrameDelay;
void Start() {
StartCoroutine(DisableAtADistance());
}
IEnumerator DisableAtADistance() {
while(true) {
float distSqrd = (transform.position - _target.transform.position).sqrMagnitude;
if (distSqrd < _maxDistance * _maxDistance) {
enabled = true;
} else {
enabled = false;
}
for (int i = 0; i < _coroutineFrameDelay; ++i) {
yield return new WaitForEndOfFrame();
}
}
}
We should assign the player's character object (or whatever object we want it to compare with) to the _target field in the Inspector window, define the maximum distance in _maxDistance, and modify the frequency with which the coroutine is invoked using the _coroutineFrameDelay field. Anytime the object goes further than _maxDistance distance away from the object assigned to _target, it will be disabled. It will be re-enabled if it returns within that distance.
A subtle performance-enhancing feature of this implementation is comparing against distance-squared instead of the raw distance. This leads us conveniently to our next section.