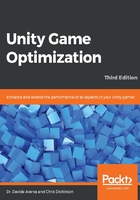
Implementing the messaging system
Let's define our messaging system by deriving from the SingletonComponent class and provide a method for objects to register with it:
using System.Collections.Generic;
using UnityEngine;
public class MessagingSystem : SingletonComponent<MessagingSystem> {
public static MessagingSystem Instance {
get { return ((MessagingSystem)_Instance); }
set { _Instance = value; }
}
private Dictionary<string,List<MessageHandlerDelegate>> _listenerDict = new Dictionary<string,List<MessageHandlerDelegate>>();
public bool AttachListener(System.Type type, MessageHandlerDelegate handler) {
if (type == null) {
Debug.Log("MessagingSystem: AttachListener failed due to having no " +
"message type specified");
return false;
}
string msgType = type.Name;
if (!_listenerDict.ContainsKey(msgType)) {
_listenerDict.Add(msgType, new List<MessageHandlerDelegate>());
}
List<MessageHandlerDelegate> listenerList = _listenerDict[msgType];
if (listenerList.Contains(handler)) {
return false; // listener already in list
}
listenerList.Add(handler);
return true;
}
}
The _listenerDict field is a dictionary of strings mapped to lists containing MessageHandlerDelegate. This dictionary organizes our listener delegates into lists by which message type they wish to listen to. Hence, if we know what message type is being sent, then we can quickly retrieve a list of all delegates that have been registered for that message type. We can then iterate through the list, querying each listener to check whether one of them wants to handle it.
The AttachListener() method requires two parameters: a message type in the form of its System.Type and MessageHandlerDelegate to send the message to when the given message type comes through the system.