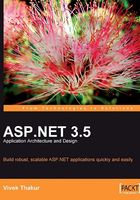
A Sample Project using Inbuilt Data Source Controls
Let's start with our good old guestbook application! This time we will see how to insert data using data controls with little or no programming. Here is how the form looks:

Open the default.aspx
form and drag an access Data Source control onto it. Put a few textbox controls on the web form, as this time we will perform insert operations without writing any SQL code. Now, we need to set the insert parameters declaratively:

This is how the code-behind will now look:
using System; using System.Data; using System.Data.OleDb; using System.Configuration; using System.Collections; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.UI.HtmlControls; namespace Chapter2.DataSource { public partial class Default : System.Web.UI.Page { protected void btnAddComment_Click(object sender, EventArgs e) { commentsDataSource.Insert(); rptComments.DataBind(); } }//end class }//end namespace
As we can see, there is absolutely no data access code except calling the insert method of the data control. Here is the ASPX where we had set the insert parameters declaratively using Visual Studio:
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="Chapter2.DataSource.Default" %> <html> <head id="Head1" runat="server"> <title>Chapter 2: Data source control sample in ASP.NET</title> </head> <body> <form id="form1" runat="server"> <div> <h1> Guestbook Entries</h1> <br /> Name: <asp:TextBox ID="txtFullName" runat="server" Width="174px"> </asp:TextBox> Email ID: <asp:TextBox ID="txtEmailID" runat="server" Width="174px"> </asp:TextBox><br /> Website: <asp:TextBox ID="txtWebsite" runat="server" Width="173px"> </asp:TextBox><br /> Comments: <asp:TextBox ID="txtComments" runat= "server" TextMode="MultiLine"></asp:TextBox><br /> <br /> <asp:Button ID="btnAddComment" runat="server" Text= "Add New Comment" OnClick="btnAddComment_Click" /><br /> <br /> <asp:AccessDataSource ID="commentsDataSource" runat= "server" DataFile="~/App_Data/Guestbook.mdb" SelectCommand="SELECT * FROM [GuestBook]" InsertCommand="INSERT INTO GuestBook(FullName, EmailID, Website, Comments) VALUES (@FullName,EmailID,Website,Comments)"> <InsertParameters> <asp:ControlParameter Name="@FullName" ControlID="txtFullName" PropertyName="Text" /> <asp:ControlParameter Name="@EmailID" ControlID="txtEmailID" PropertyName="Text" /> <asp:ControlParameter Name="@Website" ControlID="txtWebsite" PropertyName="Text" /> <asp:ControlParameter Name="@Comments" ControlID="txtComments" PropertyName="Text" /> </InsertParameters> </asp:AccessDataSource> <asp:Repeater id="rptComments" runat="server" DataSourceID= "commentsDataSource"> <ItemTemplate> Name:<%# Eval("FullName")%> <br> Email:<%# Eval("EmailID")%> <br> Website: <%# Eval("Website")%> <br> Dated: <%# Eval("EntryDate")%> <br> Comments:<%# Eval("Comments")%> </ItemTemplate> </asp:Repeater> </div> </form> </body> </html>
The use of Data Source Controls can cut down the use of data access code and shorten the development time. In the above example, we inserted the data without writing any code. Similarly, we can perform updates, deletes, and other CRUD operations, and use data controls with other controls such as the GridView and the DetailsView.
The Data Source Controls wrap the data access code logic, and use declarative markup in the ASPX page. So using these controls means that we are strongly coupling the GUI markup with the data access code, which may be fine for small applications such as a personal website, but should not be used for any commercial medium-to-large web applications.
When using data controls, the architecture is still 1-tier, but the layers change. Instead of using data fetching, and binding code in the code-behind classes, we simply use the declarative markup of Data Source Controls. This is good for turning out applications quickly, but we lose finer control over data-access.

We have all of the code, as well as the markup, in the ASPX page. As mentioned earlier, having the data access code in the GUI violates the basic principle of code separation—the GUI should be independent of the business logic or data access code. Therefore, data controls are useful for small projects only. Here is a list of the advantages and disadvantages of using data controls in web projects:
Advantages:
- Cuts down heavily on coding and saves cost and development time.
- Declarative markup allows changes to propagate on the server without re-compiling the site.
- Ready support for data-bound controls such as GridView, DetailsView, and DataList.
Disadvantages:
- Highly-coupled GUI and data access code.
- Only good for small applications with no business logic.
- Scalability issues—if the project grows big, then performance will be affected.
- Not efficient when dealing with complex hierarchical result-sets.
- Because they abstract data access operations, they are not very flexible and may present problems when dealing with customized data.
A simple guestbook application for a personal website is one such case warranting the use of data controls. Such applications have quick turn-around time, and one can build a working application in a matter of few days. If we do not use Data Source Controls, then we can directly write custom data access code in the code-behind file. Although we will be putting non-UI related code in the UI layer even then, we will have more flexibility as we can write custom SQL statements and modify data at runtime, which is quite difficult to do using Data Source Controls.
To overcome the disadvantages of data controls, Microsoft introduced Object Data Source control, to support business objects, and business logic code and the N-tier architecture. We will learn more about object Data Source Controls in the next chapter.