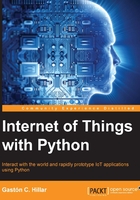
Turning on and off an onboard component
First, we will take advantage of an onboard LED (Light Emitting Diode) to write our first Python lines that interact with the digital output capabilities included in the Intel Galileo Gen 2 board. The simple example will allow us to understand how the mraa
library allows us to easily turn on and off one of the onboard components with Python code.
In the previous chapter, we recognized the different elements included in the Intel Galileo Gen 2 board. We know that there are three rectangular LEDs located at the right hand side of the USB 2.0 host connector. The first LED, labeled L is connected to pin 13 of the digital I/O pins, and therefore, a high level sent to pin 13 will turn on this LED and a low level will turn it off.
We will write a few lines of Python code that will use the mraa
library to make the onboard LED labeled L to repeat the following loop until the Python program is interrupted:
- Turn on
- Stay turned on for 3 seconds
- Turn off
- Stay turned off for 2 seconds.
The following lines show the Python code that performs the previously explained actions. The code file for the sample is iot_python_chapter_03_01.py
.
import mraa import time if __name__ == "__main__": print ("Mraa library version: {0}".format(mraa.getVersion())) print ("Mraa detected platform name: {0}".format(mraa.getPlatformName())) # Configure GPIO pin #13 to be an output pin onboard_led = mraa.Gpio(13) onboard_led.dir(mraa.DIR_OUT) while True: # Turn on the onboard LED onboard_led.write(1) print("I've turned on the onboard LED.") # Sleep 3 seconds time.sleep(3) # Turn off the onboard LED onboard_led.write(0) print("I've turned off the onboard LED.") time.sleep(2)
Tip
Detailed steps to download the code bundle are mentioned in the Preface of this book. Please have a look.
The code bundle for the book is also hosted on GitHub at https://github.com/PacktPublishing/Internet-of-Things-with-Python. We also have other code bundles from our rich catalog of books and videos available at https://github.com/PacktPublishing/. Check them out!
In the previous chapter, we learned that the Yocto Linux running on the board provided both SSH and SFTP (short for SSH File Transfer Protocol or Secure File Transfer Protocol) services by running a Bonjour browser. We can use any SFTP client to connect to the board and transfer the file that we created in any computer or mobile device. Of course, we can also use any Linux editor, such as vi
, in the SSH terminal, or just enter the code in the Python interpreter. However, it is usually more convenient to use our favorite editor or IDE in our computer or mobile device and then transfer the file to the board with any SFTP client.
We don't want the process to be linked to a specific IDE, and therefore, we will transfer the file with an SFTP client. FileZilla Client is a free, open source and multiplatform FTP client that supports SFTP. You can download and install it here: http://filezilla-project.org.
Once you have installed and executed FileZilla Client, you must follow the next steps to add the SFTP server running on the board in with the application's Site Manager:
- Select File | Site Manager.
- Click New Site on the Site Manager dialog box. Enter the desired name, such as IntelGalileo2 to easily identify the board's SFTP service.
- Enter the board's IP address in Host. You don't need to enter any value in Port because the SFTP server uses the default SFTP port, that is, the same port in which the SSH daemon listens: port 22.
- Select SFTP - SSH File Transfer Protocol in the Protocol dropdown.
- Select Normal in the Logon Type dropdown.
- Enter root in User. The next screenshots shows the configuration values for a board that has 192.168.1.107 as its assigned IP address.
- Click Connect. FileZilla will display an Unknown host key dialog box, indicating that the server's host key is unknown. It is similar to the information provided when you established the first connection to the board with an SSH client. The details include the host and the fingerprint. Activate the Always trust this host, add this key to the cache checkbox and click OK.
- FileZilla will display the
/home/root
folder for the Yocto Linux running on the board at the right-hand side of the window, under Remote Site. - Navigate to the folder in which you saved the Python files you want to transfer in your local computer under Local site.
- Select the file you want to transfer and press Enter to transfer the file to the
/home/root
folder on the board. Another way is to right-click on the desired file and select Upload. FileZilla will display the uploaded file in the/home/root
folder under Remote Site. This way, you will be able to access the Python file in the default location that Yocto Linux uses when you login with an SSH terminal, that is, in your home folder for yourroot
user. The following picture shows many Python files uploaded to the/home/root
folder with FileZilla and listed in the contents of the/home/root
folder.
The next time you have to upload a file to the board, you don't need to setup a new site in the Site Manager dialog box in order to establish an SFTP connection. You just need to select File | Site Manager, select the site name under Select Entry and click Connect.
If you run the following command in the SSH terminal after you login, Linux will print your current folder or directory:
pwd
The result of the previous command will be the same folder in which we uploaded the Python code file.
/home/root
Once we transfer the file to the board, we can run the previous code with the following command on the board's SSH terminal:
python iot_python_chapter_03_01.py
The previous code is extremely simple. We have used many print statements to make it easy for us to understand what is going on with messages on the console. The following lines show the generated output after we run the code for a few seconds:
Mraa library version: v0.9.0 Mraa detected platform name: Intel Galileo Gen 2 Setting GPIO Pin #13 to dir DIR_OUT I've turned on the onboard LED. I've turned off the onboard LED. I've turned on the onboard LED. I've turned off the onboard LED.
The first lines print the mraa
library version and the detected platform name. This way, we have information about the mraa
library version that Python is using and we make sure that the mraa
library has been able to initialize itself and detect the right platform: Intel Galileo Gen 2. In case we have a specific issue, we can use this information to check about specific problems related to the mraa
library and the detected platform.
The next line creates an instance of the mraa.Gpio
class. GPIO stands for General Purpose Input/Output and an instance of the mraa.Gpio
class represents a general purpose Input/Output pin on the board. In this case, we pass 13
as an argument for the pin
parameter, and therefore, we are creating an instance of the mraa.Gpio
class that represents the pin number 13 of the GPIO pins in the board. We named the instance onboard_led
to make it easy to understand that the instance allows us to control the status of the onboard LED.
onboard_led = mraa.Gpio(13)
Tip
We just need to specify the value for the pin parameter to initialize an instance of the mraa.Gpio
class. There are two additional optional parameters (owner
and raw
), but we should leave them with the default values. By default, whenever we create an instance of the mraa.Gpio
class, we own the pin and the mraa
library will close it on destruct.
As we might guess from its name, an instance of the mraa.Gpio
class allows us to work with pins as either Input or Output. Thus, it is necessary to specify the desired direction for our mraa.Gpio
instance. In this case, we want to use pin 13 as an output pin. The following line calls the dir
method to configure the pin to be an output pin, that is, to set is direction to the mraa.DIR_OUT
value.
onboard_led.dir(mraa.DIR_OUT)
Then, the code runs a loop forever, that is, until you interrupt the execution by pressing Ctrl + C or the button to stop the process in case you are using a Python IDE with remote development features to run the code in your board.
The first line within the while
loop calls the write
method for the mraa.Gpio
instance, onboard_led
, with 1
as an argument for the value
required parameter. This way, we send a high value (1
) to the pin 13 configured for digital output. Because the pin 13 has the onboard LED connected to it, the result of a high value in pin 13 is that the onboard LED turns on.
onboard_led.write(1)
After we turn on the LED, a line of code uses the print
statement to print a message to the console output, so that we know the LED should be turned on. A call to time.sleep
with 3
as the value for the seconds
argument delays the execution for three seconds. Because we didn't change the status of pin 13, the LED will stay turned on during this delay.
time.sleep(3)
The next line calls the write
method for the mraa.Gpio
instance, onboard_led
, but this time with 0
as an argument for the value
required parameter. This way, we send a low value (0
) to the pin 13 configured for digital output. Because the pin 13 has the onboard LED connected to it, the result of a low value in pin 13 is that the onboard LED turns off.
onboard_led.write(0)
After we turn off the LED, a line of code uses the print
statement to print a message to the console output, so that we know the LED should be turned off. A call to time.sleep
with 2
as the value for the seconds argument delays the execution for 2 seconds. Because we didn't change the status of pin 13, the LED will stay turned off during this delay. Then, the loop starts over again.
Tip
As we can use any ssh
client to run the Python code, we can see the results of the print
statements in the console output and they are extremely useful for us to understand what should be happening with the digital outputs. We will take advantage of more advanced logging features included in Python for more complex scenarios later.
As we could learn from the previous example, the mraa
library encapsulates all the necessary methods to work with the GPIO pins in the mraa.Gpio
class. The previous code didn't take advantage of Python's object-oriented features, it just interacted with one of the classes included in the mraa
library. We will take advatange of many Python features in the forthcoming examples. In addition, once we start working with more complex examples, we will make the board interact through the network.